Survey Data Conversion¶
Get survey data from OGP measurement files
- hps_align.survey.data(year: int = <typer.models.ArgumentInfo object>, input_file: ~pathlib.Path = <typer.models.ArgumentInfo object>, output_file: str = <typer.models.OptionInfo object>)¶
some more explanation
Coordinate Systems¶
To include the survey measurements in the alignment, they need to be converted to the correct coordinate systems. The following classes and functions are used to do this.
The Uchannel has two coordinate systems, the ball frame and the pin frame which differ for top and bottom, see images below.
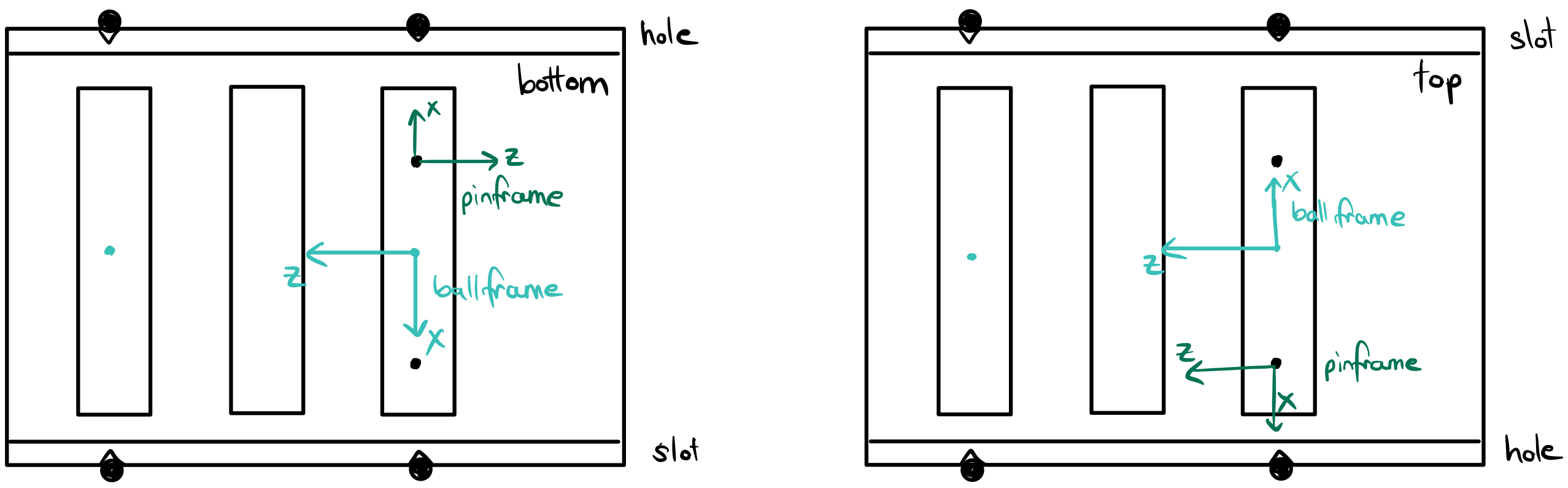
Ball Frame¶
- class hps_align.survey._ballframe.BallFrame(input_file=None)¶
Bases:
object
SVT uchannel ball frame class
Gets ball positions from survey data file and calculates basis vectors and origin. The ball positions in the survey data file should be given in the OGP (or other global) system.
- L1_hole_ball_dict¶
Dictionary of L1 hole ball coordinates {‘x’: x, ‘y’: y, ‘z’: z}
- Type:
dict
- L1_slot_ball_dict¶
Dictionary of L1 slot ball coordinates {‘x’: x, ‘y’: y, ‘z’: z}
- Type:
dict
- L3_hole_ball_dict¶
Dictionary of L3 hole ball coordinates {‘x’: x, ‘y’: y, ‘z’: z}
- Type:
dict
- L3_slot_ball_dict¶
Dictionary of L3 slot ball coordinates {‘x’: x, ‘y’: y, ‘z’: z}
- Type:
dict
- get_ball(layer, ball_type)¶
Get ball coordinates for a given layer and ball type
The coordinates are either read from survey data file or have been set manually before.
- Parameters:
layer (int) – Layer number
ball_type (str) – Ball type (‘hole’ or ‘slot’)
- Returns:
ball – Ball position in OGP (or other global) coordinates
- Return type:
np.array
- get_basis(volume='')¶
Get ballframe basis vectors
- Returns:
basis (np.array) – Basis vectors in OGP (or other global) coordinates
origin (np.array) – Origin coordinates in OGP (or other global) coordinates
- get_midpoint(layer)¶
Get midpoint between hole and slot balls for a given layer
- Parameters:
layer (int) – Layer number
- Returns:
midpoint – Position of midpoint in OGP (or other global) coordinates
- Return type:
np.array
- set_ball(ball_coords, layer, ball_type)¶
Set ball coordinates for a given layer and ball type
Overwrites the ball coordinates from the survey data file.
- Parameters:
ball_coords (dict) – Dictionary of ball coordinates {‘x’: x, ‘y’: y, ‘z’: z}
layer (int) – Layer number
ball_type (str) – Ball type (‘hole’ or ‘slot’)
Pin Frame¶
To construct the pin frame, the base plane and pins are needed.
Base Planes¶
- class hps_align.survey._baseplanes.BasePlane(input_file=None)¶
Bases:
object
SVT uchannel base plane class
The base planes are at the locations at which the sensors are mounted. The pins are mounted on the base planes. The base plane coordinates in the survey data file should be given in the OGP (or other global) system.
- L0_base_plane_dict¶
Dictionary of L0 base plane coordinates {‘x’: x, ‘y’: y, ‘z’: z, ‘xy_angle’: xy_angle, ‘elevation’: elevation}
- Type:
dict
- L1_base_plane_dict¶
Dictionary of L1 base plane coordinates {‘x’: x, ‘y’: y, ‘z’: z, ‘xy_angle’: xy_angle, ‘elevation’: elevation}
- Type:
dict
- L2_base_plane_dict¶
Dictionary of L2 base plane coordinates {‘x’: x, ‘y’: y, ‘z’: z, ‘xy_angle’: xy_angle, ‘elevation’: elevation}
- Type:
dict
- L3_base_plane_dict¶
Dictionary of L3 base plane coordinates {‘x’: x, ‘y’: y, ‘z’: z, ‘xy_angle’: xy_angle, ‘elevation’: elevation}
- Type:
dict
- get_base_plane(layer)¶
Get base plane origin coordinates and normal vector for a given layer
The coordinates are either read from survey data file or have been set manually before.
- Parameters:
layer (int) – Layer number
- Returns:
origin (np.array) – Base plane origin coordinates
normal (np.array) – Base plane normal vector
- set_base_plane_dict(base_plane_coords, layer)¶
Set base plane coordinates for a given layer
Overwrites the pin coordinates from the survey data file.
- Parameters:
base_plane_coords (dict) – Dictionary of base plane coordinates
layer (int) – Layer number
Pins¶
- class hps_align.survey._pins.Pin(input_file=None)¶
Bases:
object
SVT uchannel pin class
The pins are mounted on the base planes and are used to align the sensors. The pin coordinates in the survey data file should be given in the OGP (or other global) system.
- L0_hole_pin_dict¶
Dictionary of L0 hole pin coordinates {‘x’: x, ‘y’: y, ‘z’: z}
- Type:
dict
- L0_slot_pin_dict¶
Dictionary of L0 slot pin coordinates {‘x’: x, ‘y’: y, ‘z’: z}
- Type:
dict
- L1_hole_pin_dict¶
Dictionary of L1 hole pin coordinates {‘x’: x, ‘y’: y, ‘z’: z}
- Type:
dict
- L1_slot_pin_dict¶
Dictionary of L1 slot pin coordinates {‘x’: x, ‘y’: y, ‘z’: z}
- Type:
dict
- L2_hole_pin_dict¶
Dictionary of L2 hole pin coordinates {‘x’: x, ‘y’: y, ‘z’: z}
- Type:
dict
- L2_slot_pin_dict¶
Dictionary of L2 slot pin coordinates {‘x’: x, ‘y’: y, ‘z’: z}
- Type:
dict
- L3_hole_pin_dict¶
Dictionary of L3 hole pin coordinates {‘x’: x, ‘y’: y, ‘z’: z}
- Type:
dict
- L3_slot_pin_dict¶
Dictionary of L3 slot pin coordinates {‘x’: x, ‘y’: y, ‘z’: z}
- Type:
dict
- get_pin(layer, pin_type)¶
Get pin coordinates
The coordinates are either read from survey data file or have been set manually before.
- Parameters:
layer (int) – Layer number
pin_type (str) – Pin type
- Returns:
pin – Pin position in OGP (or other global) coordinates
- Return type:
np.array
- set_pin(pin_coords, layer, pin_type)¶
Set pin coordinates to given values
Overwrites the pin coordinates from the survey data file.
- Parameters:
pin_coords (dict) – Dictionary of pin coordinates {‘x’: x, ‘y’: y, ‘z’: z}
layer (int) – Layer number
pin_type (str) – Pin type
Pin Frame¶
- class hps_align.survey._pinframe.PinFrame(input_file=None)¶
Bases:
object
SVT uchannel pin frame class
Gets pin positions from survey data file and calculates basis vectors and origin using the BasePlane and Pin classes.
- input_file¶
Survey data file
- Type:
str
- get_basis(layer)¶
Get basis vectors for pin frame
- Parameters:
layer (int) – Layer number
- Returns:
basis (np.array) – Basis vectors in OGP (or other global) coordinates
hole_pin_projected (np.array) – Origin in OGP (or other global) coordinates
UChannel¶
- class hps_align.survey._uchannel.UChannel(ballframe_top=<hps_align.survey._ballframe.BallFrame object>, ballframe_bot=<hps_align.survey._ballframe.BallFrame object>, pinframe_top=<hps_align.survey._pinframe.PinFrame object>, pinframe_bot=<hps_align.survey._pinframe.PinFrame object>)¶
Bases:
object
SVT uchannel class
The uchannel has two volumes, top and bottom. Each volume has a ball frame and multiple pin frames. The PinFrame class returns the pin basis for each layer. This class is used to calculate the pin frame basis in the uchannel ball frame.
- get_ball_basis(volume)¶
Get basis vectors and origin for ball frame
There is only one ball frame per volume.
- Parameters:
volume (str) – Volume (‘top’ or ‘bottom’)
- Returns:
basis (np.array) – Numpy array of basis vectors
origin (np.array) – Numpy array of origin coordinates
- get_pin_basis(layer, volume)¶
Get basis vectors and origin for pin frame
There is a pin frame for each layer that is determined by the respective positions of the pins.
- Parameters:
layer (int) – Layer number
volume (str) – Volume (‘top’ or ‘bottom’)
- Returns:
basis (np.array) – Numpy array of basis vectors
origin (np.array) – Numpy array of origin coordinates
- pin_in_ballframe(layer, volume)¶
Transform pin coordinates to ball frame coordinates
- Parameters:
layer (int) – Layer number
volume (str) – Volume (‘top’ or ‘bottom’)
- Returns:
pin_to_ball – Numpy array of pin coordinates in ball frame
- Return type:
np.array
Fixture¶
The fixture also has a pin and a ball frame, see image below. It is important to note that the pin frame is analogous to the pin frame of the UChannel, but the ball frame is different.
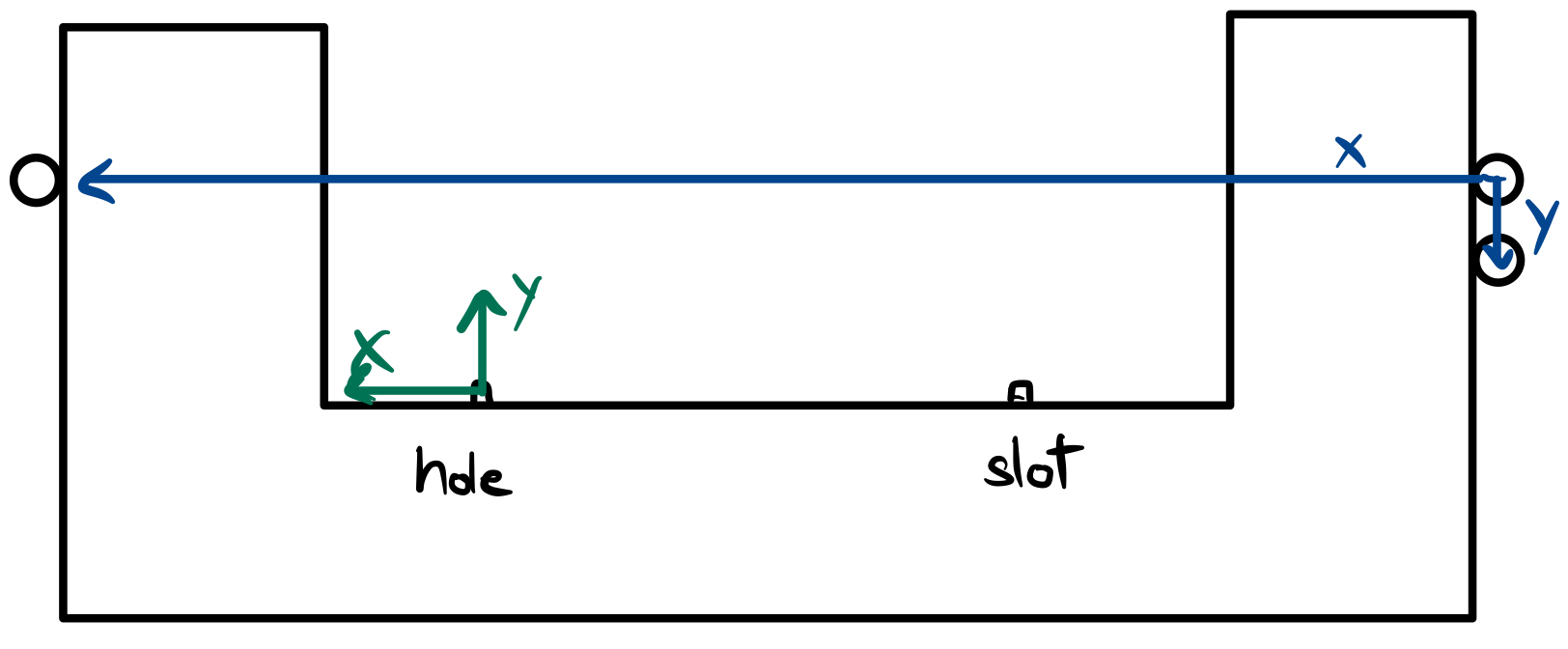
- class hps_align.survey._fixture.Fixture(input_file=None)¶
Bases:
object
Fixture for measuring sensors
The fixture is used to measure the sensors. It consists of a base plane, a ball frame and a pin frame. The pins are used to align the sensors and have the same distance as the uchannel pins. Additionally, the pin frame in the fixture is defined analogously to the uchannel pin frame. The pin positions cannot be used as a reference for meaurement once the sensor is installed. The fixture ball frame is used as a reference frame for the sensor measurements. Importantly, the fixture ball frame is not the same as the uchannel ball frame. Here, the ball frame is defined by three balls on the outside of the fixture.
All measurements should be in the same global coordinate system, e.g. OGP.
- oriball_dict¶
Dictionary of fixture ball coordinates {‘x’: x, ‘y’: y, ‘z’: z}, origin of ball frame
- Type:
dict
- diagball_dict¶
Dictionary of fixture ball coordinates {‘x’: x, ‘y’: y, ‘z’: z}
- Type:
dict
- axiball_dict¶
Dictionary of fixture ball coordinates {‘x’: x, ‘y’: y, ‘z’: z}
- Type:
dict
- ball_plane_dict¶
Dictionary of fixture ball coordinates {‘x’: x, ‘y’: y, ‘z’: z, ‘xy_angle’: xy_angle, ‘elevation’: elevation}
- Type:
dict
- base_plane_dict¶
Dictionary of base plane coordinates {‘x’: x, ‘y’: y, ‘z’: z, ‘xy_angle’: xy_angle, ‘elevation’: elevation}
- Type:
dict
- oripin_dict¶
Dictionary of pin coordinates {‘x’: x, ‘y’: y, ‘z’: z}, slot side
- Type:
dict
- axipin_dict¶
Dictionary of pin coordinates {‘x’: x, ‘y’: y, ‘z’: z}, hole side
- Type:
dict
- get_ball(type)¶
Get fixture ball coordinates from survey data file
The coordinates are either read from survey data file or have been set manually before.
- Parameters:
type (str) – Type of ball to get coordinates for
- Returns:
ball – Ball position in OGP (or other global) coordinates
- Return type:
np.array
- get_ball_basis()¶
Get basis vectors for fixture
- Returns:
basis (np.array) – Basis vectors in OGP (or other global) coordinates
origin (np.array) – Origin in OGP (or other global) coordinates
- get_ball_in_pin()¶
- get_base_plane()¶
Get base plane coordinates.
The coordinates are either read from survey data file or have been set manually before.
- Returns:
origin (np.array) – Base plane origin in OGP (or other global) coordinates
normal (np.array) – Normal vector of base plane in OGP (or other global) coordinates
- get_pin(type)¶
Get pin coordinates.
The coordinates are either read from survey data file or have been set manually before.
- Parameters:
type (str) – Type of pin to get coordinates for
- Returns:
pin – Pin position in OGP (or other global) coordinates
- Return type:
np.array
- get_pin_basis()¶
Get basis vectors for pin frame
- Returns:
basis (np.array) – Basis vectors in OGP (or other global) coordinates
origin (np.array) – Origin in OGP (or other global) coordinates
- get_pin_in_ball()¶
Get basis vectors for pin frame in fixture ball coordinates
- Returns:
basis (np.array) – Basis vectors in fixture ball frame
origin (np.array) – Origin in fixture ball frame
- set_ball(ball_coords, type)¶
Set fixture ball coordinates for a given layer and ball type
Overwrites the fixture ball coordinates from the survey data file.
- Parameters:
ball_coords (dict) – Dictionary of ball coordinates
type (str) – Type of ball to set coordinates for
- set_base_plane(base_plane_coords)¶
Set base plane coordinates
Overwrites the base plane coordinates from the survey data file.
- Parameters:
base_plane_coords (dict) – Dictionary of base plane coordinates
- set_pin(pin_coords, type)¶
Set pin coordinates for a given layer and pin type
Overwrites the pin coordinates from the survey data file.
- Parameters:
pin_coords (dict) – Dictionary of pin coordinates
type (str) – Type of pin to set coordinates for
Sensors¶
The sensor-local coordinates are labeled UVW and are different from the global XYZ coordinates by a translation and rotation. The coordinate systems are different in the front and back as well as top and bottom of the SVT, see images below.
Front¶
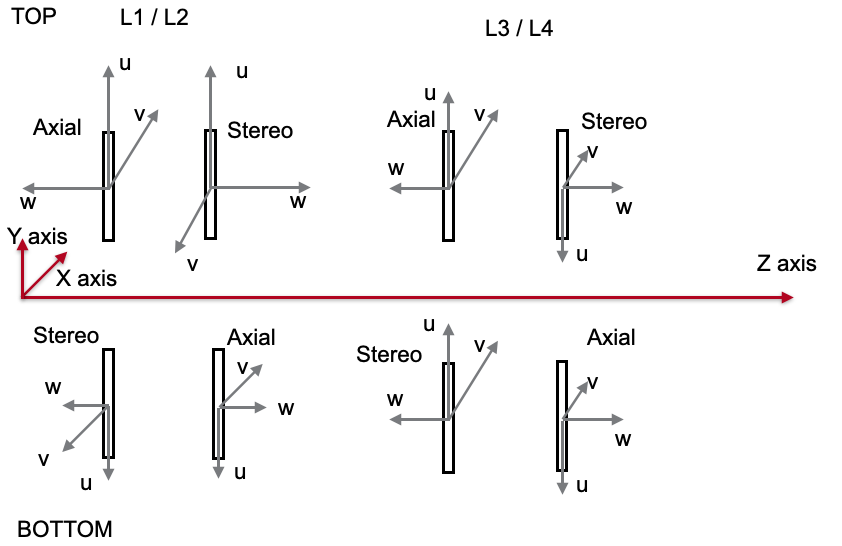
Back¶
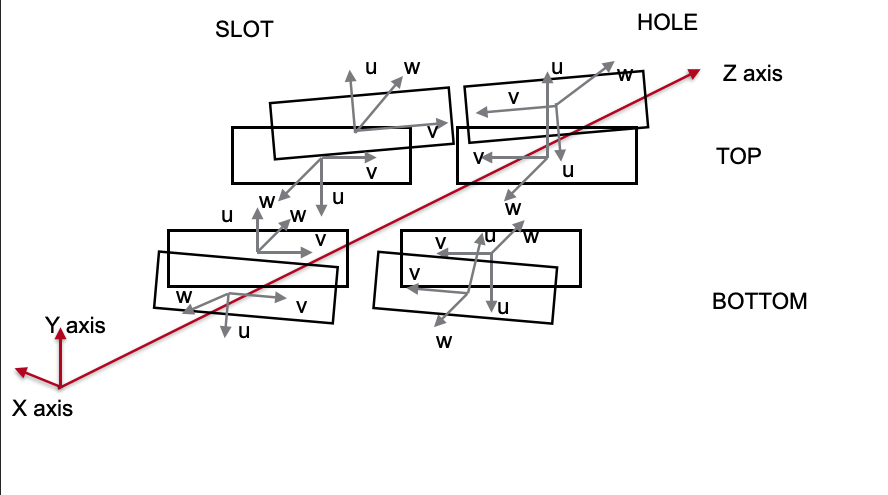
- class hps_align.survey._sensors.Sensor(fixture, input_file=None)¶
Bases:
object
SVT sensor class
The sensors are measured while mounted in the fixture. In addition to the sensor’s position and orientation, the position of the ficxture balls has to be measured. The sensor coordinates in the survey data file should be given in the OGP (or other global) system.
- oriball_dict¶
Dictionary of ball coordinates {‘x’: x, ‘y’: y, ‘z’: z}, origin of ball frame
- Type:
dict
- diagball_dict¶
Dictionary of ball coordinates {‘x’: x, ‘y’: y, ‘z’: z}
- Type:
dict
- axiball_dict¶
Dictionary of ball coordinates {‘x’: x, ‘y’: y, ‘z’: z}
- Type:
dict
- ball_plane_dict¶
Dictionary of ball plane coordinates {‘x’: x, ‘y’: y, ‘z’: z, ‘xy_angle’: xy_angle, ‘elevation’: elevation}
- Type:
dict
- sensor_plane_dict¶
Dictionary of sensor plane coordinates {‘x’: x, ‘y’: y, ‘z’: z, ‘xy_angle’: xy_angle, ‘elevation’: elevation}
- Type:
dict
- sensor_origin_dict¶
Dictionary of sensor origin coordinates {‘x’: x, ‘y’: y, ‘z’: z}
- Type:
dict
- get_ball(balltype)¶
Get ball coordinates from survey data file
- Parameters:
balltype (str) – Type of ball to get coordinates for
- Returns:
ball – Fixture ball position in OGP (or other global) coordinates
- Return type:
np.array
- get_ball_basis()¶
Get ball basis
- Returns:
basis (np.array) – Fixture ball basis vectors in OGP (or other global) coordinates
origin (np.array) – Origin in OGP (or other global) coordinates
- get_sensor_normal()¶
Get sensor normal vector
- Returns:
normal – Normal vector to sensor plane in OGP (or other global) coordinates
- Return type:
np.array
- get_sensor_normal_ballframe()¶
Get sensor normal coordinates in fixture ballframe
- Returns:
normal – Sensor normal vector in fixture ballframe
- Return type:
np.array
- get_sensor_normal_pinframe()¶
Get sensor normal vector in pinframe
- Returns:
normal – Sensor normal vector in fixture pinframe
- Return type:
np.array
- get_sensor_origin()¶
Get sensor origin coordinates
- Returns:
origin – Sensor origin in OGP (or other global) coordinates
- Return type:
np.array
- get_sensor_origin_ballframe()¶
Get sensor origin coordinates in fixture ballframe
- Returns:
origin – Sensor origin coordinates in fixture ballframe
- Return type:
np.array
- get_sensor_origin_pinframe()¶
Get sensor origin coordinates in pinframe
- Returns:
origin – Sensor origin in fixture pinframe
- Return type:
np.array
- set_ball(ball_coords, balltype)¶
Set ball coordinates for a given layer and ball type
Overwrites the ball coordinates from the survey data file.
- Parameters:
ball_coords (dict) – Dictionary of ball coordinates
type (str) – Type of ball to set coordinates for
- set_sensor_origin(sensor_origin)¶
Set sensor origin coordinates
Overwrites the sensor origin coordinates from the survey data file.
- Parameters:
sensor_origin (dict) – Dictionary of sensor origin coordinates
- set_sensor_plane(sensor_plane)¶
Set sensor plane coordinates
Overwrites the sensor plane coordinates from the survey data file.
- Parameters:
sensor_plane (dict) – Dictionary of sensor plane coordinates
Special Coordinate Systems¶
In the past, the coordinate system has occasionally been changed during the measurement of the survey data. The following classes and functions are used to convert the data from these special coordinate systems.
Matt Ball Frame¶
Special ball frame class for Matt Solt’s measurement data.
- class hps_align.survey._ballframe.MattBallFrame(input_file=None)¶
Bases:
BallFrame
Ballframe class for Matt’s survey measurements.
The coordinate system was changed during the measurement. Ball positions and midpoints are given in the OGP system, but the pin positions, that have been measured in the same measurement, are given in a new system. To get the correct relation between the ballframe and the pinframe, the ballframe basis is returned in the new system (‘Matt system’) and not in the OGP system.
- L1_midpoint_dict¶
Dictionary of L1 midpoint coordinates {‘x’: x, ‘y’: y, ‘z’: z}
- Type:
dict
- L3_midpoint_dict¶
Dictionary of L3 midpoint coordinates {‘x’: x, ‘y’: y, ‘z’: z}
- Type:
dict
- get_basis(volume)¶
Get ballframe basis vectors in Matt system
- Parameters:
volume (str) – ‘top’ or ‘bottom’
- Returns:
basis (np.array) – Basis vectors in Matt coordinates
origin (np.array) – Origin in Matt coordinates
- get_matt_basis(volume)¶
Get basis vectors for Matt’s ballframe
- Parameters:
volume (str) – ‘top’ or ‘bottom’
- Returns:
basis (np.array) – Basis vectors in OGP (or other global) coordinates
origin (np.array) – Origin in OGP (or other global) coordinates
- get_meas_midpoint(layer)¶
Get midpoint coordinates for a given layer
- Parameters:
layer (int) – Layer number
- Returns:
midpoint – Midpoint position in OGP (or other global) coordinates
- Return type:
np.array
- set_midpoint(midpoint_coords, layer)¶
Set midpoint coordinates for a given layer
Overwrites the midpoint coordinates from the survey data file.
- Parameters:
midpoint_coords (dict) – Dictionary of midpoint coordinates {‘x’: x, ‘y’: y, ‘z’: z}
layer (int) – Layer number
Matt Fixture¶
Special fixture class to use Matt Solt’s measurement data.
Matt Sensor¶
Special sensor class to use Matt Solt’s measurement data.
- class hps_align.survey._mattsensor.MattSensor(fixture, input_file=None)¶
Bases:
Sensor
SVT sensor class for Matt’s survey measurement
Matt changed the coordinate system during the measurement. The ball positions are in OGP coordinates, the sensor positions are in the new coordinate system (‘Matt system’).
- oriball_dict¶
Dictionary of ball coordinates {‘x’: x, ‘y’: y, ‘z’: z}, origin of ball frame, OGP coordinates
- Type:
dict
- diagball_dict¶
Dictionary of ball coordinates {‘x’: x, ‘y’: y, ‘z’: z}, OGP coordinates
- Type:
dict
- axiball_dict¶
Dictionary of ball coordinates {‘x’: x, ‘y’: y, ‘z’: z}, OGP coordinates
- Type:
dict
- ball_plane_dict¶
Dictionary of ball plane coordinates {‘x’: x, ‘y’: y, ‘z’: z, ‘xy_angle’: xy_angle, ‘elevation’: elevation}, Matt coordinates
- Type:
dict
- sensor_origin_dict¶
Dictionary of sensor origin coordinates {‘x’: x, ‘y’: y, ‘z’: z}, Matt coordinates
- Type:
dict
- sensor_plane_dict¶
Dictionary of sensor plane coordinates {‘x’: x, ‘y’: y, ‘z’: z, ‘xy_angle’: xy_angle, ‘elevation’: elevation}, Matt coordinates
- Type:
dict
- _find_sensor_active_edge_away()¶
Find sensor active edge (away from beam) coordinates in survey data file
- Returns:
sensor_active_edge – Dictionary of sensor active edge coordinates in Matt coordinates
- Return type:
dict
- _find_sensor_active_edge_beam()¶
Find sensor active edge (closer to beam) coordinates in survey data file
- Returns:
sensor_active_edge – Dictionary of sensor active edge coordinates in Matt coordinates
- Return type:
dict
- _find_sensor_physical_edge()¶
Find sensor physical edge coordinates in survey data file
- Returns:
sensor_physical_edge – Dictionary of sensor physical edge coordinates in Matt coordinates
- Return type:
dict
- get_active_edge_dir()¶
Get sensor active edge direction in matt coordinates
- Returns:
direction – Sensor active edge direction in Matt coordinates
- Return type:
np.array
- get_matt_basis()¶
Get basis vectors for Matt sensor
- Returns:
basis (np.array) – Basis vectors in OGP (or other global) coordinates
origin (np.array) – Origin in OGP (or other global) coordinates
- get_sensor_basis_pinframe()¶
Get sensor basis vectors in fixture pinframe
- Returns:
basis (np.array) – Sensor basis vectors in fixture pinframe
origin (np.array) – Sensor origin in fixture pinframe
- get_sensor_normal()¶
Get sensor normal vector
- Returns:
normal – Normal vector to sensor plane in Matt coordinates
- Return type:
np.array
- get_sensor_normal_ballframe()¶
Get sensor normal in fixture ballframe
- Returns:
normal – Sensor normal in fixture ballframe
- Return type:
np.array
- get_sensor_origin()¶
Get sensor origin coordinates
- Returns:
origin – Sensor origin in Matt coordinates
- Return type:
np.array
- get_sensor_origin_ballframe()¶
Get sensor origin coordinates in fixture ballframe
- Returns:
origin – Sensor origin in fixture ballframe
- Return type:
np.array
- get_strip_direction_ballframe()¶
Get sensor strip direction in fixture ballframe
- Returns:
direction – Sensor strip direction in fixture ballframe
- Return type:
np.array
- get_strip_direction_pinframe()¶
Get sensor strip direction in fixture pinframe
- Returns:
direction – Sensor strip direction in fixture pinframe
- Return type:
np.array
- matt_to_ball()¶
Get transformation matrix from Matt to fixture ballframe
This is just a rotation, no translation.
- Returns:
transformation – Transformation matrix from Matt coordinates to fixture ballframe
- Return type:
np.array
Survey Data¶
Using the different reference frames, the survey classes convert the measurement data and print the results in the correct format for hps-java alignment constants.
- class hps_align.survey._survey.Survey¶
Bases:
object
SVT survey class
This class combines the UChannel and sensor measurements. Depending on the configuration of the SVT, this class contains a different set of sensors.
- sensors¶
Dictionary of sensors {volume: {layer: {sensor (ax/st): Sensor()}}}
- Type:
dict
- get_pin_in_uchannel_ballframe(volume, layer)¶
- set_sensors(sensors)¶
- set_uchannel(uchannel)¶
- transform_sensor_to_uchannel_ballframe(volume, layer, sensor_type)¶
- class hps_align.survey._survey.Survey2019(survey_files)¶
Bases:
Survey
SVT survey class for 2019 configuration
Here, only the first two layers in top and bottom are used.
- get_pin_in_uchannel_ballframe(volume, layer)¶
Get pin frame basis vectors and origin in uchannel ball frame
To attach the L2 slim sensors a transition plate is used. This transition plate has a different pin frame (small pin frame) than the original L2 uchannel pins (wide pin frame). To account for this, additional coordinate transformations are needed. For L1, the uchannel pin frame is already the small pin frame, hence no transformation is needed.
- Parameters:
volume (str) – Volume (‘top’ or ‘bottom’)
layer (int) – Layer number
- Returns:
basis (np.array) – Basis vectors in uchannel ball frame
origin (np.array) – Origin in uchannel ball frame
- print_results(out_name)¶
Print results to file
Writes pin frame basis vectors and origins in uchannel ball frame and sensor basis vectors and origins in pin frame to file.
- Parameters:
out_name (str) – Output file name
- transform_sensor_to_uchannel_ballframe(volume, layer, sensor_type)¶
Utility Functions¶
Parser¶
The parser is used to read the survey data from the files. .. automodule:: hps_align.survey._parser
- members:
- undoc-members:
- show-inheritance:
- private-members:
Utils¶
- hps_align.survey._utils.make_basis(vec1, vec2)¶
Make orthonormal basis from two vectors
- Parameters:
vec1 (np.array) – First vector, will be normalized
vec2 (np.array) – Second vector, will be orthogonalized with respect to vec1, then normalized
- Returns:
basis – Orthonormal basis
- Return type:
np.array
- hps_align.survey._utils.normal_vector(az, el)¶
Return normal vector from azimuth and elevation angles
- Parameters:
az (float) – Azimuth angle in radians
el (float) – Elevation angle in radians
- Returns:
normal – Normal vector
- Return type:
np.array
- hps_align.survey._utils.normalize(vec)¶
Normalize a vector
- hps_align.survey._utils.orthogonalize(vec1, vec2)¶
Orthogonalize vec2 with respect to vec1
- hps_align.survey._utils.project_to_plane(vec, plane_origin, plane_normal)¶
Project a vector onto a plane